Google uses cookies to deliver its services, to personalize ads, and to analyze traffic. You can adjust your privacy controls anytime in your Google settings . Learn more .
- Install Flutter
- Write your first app
- Flutter for Android devs
- Flutter for SwiftUI devs
- Flutter for UIKit devs
- Flutter for React Native devs
- Flutter for web devs
- Flutter for Xamarin.Forms devs
- Introduction to declarative UI
- Flutter versus Swift concurrency
- Dart language overview
- SDK archive
- Release notes
- Breaking changes
- Compatibility policy
- Samples and demos
- Add achievements and leaderboards
- Add advertising
- Add multiplayer support
- Add in-app purchases
- Add user authentication
- Debug using Crashlytics
- Build a news app
- Add maps to your app
- User interface
- Introduction
- Widget catalog
- Build a layout
- Create and use lists
- Create a horizontal list
- Create a grid view
- Create lists with different types of items
- Create lists with spaced items
- Work with long lists
- Use slivers to achieve fancy scrolling
- Place a floating app bar above a list
- Create a scrolling parallax effect
- Adaptive and responsive app design
- Build an adaptive app
- Update app UI based on orientation
- Share styles with themes
- Material design
- Migrate to Material 3
- Fonts & typography
- Use a custom font
- Export fonts from a package
- Google Fonts package
- Use custom fragment shaders
- Add interactivity to your app
- Handle taps
- Drag an object outside an app
- Drag a UI element within an app
- Add Material touch ripples
- Implement swipe to dismiss
- Create and style a text field
- Retrieve the value of a text field
- Handle changes to a text field
- Manage focus in text fields
- Build a form with validation
- Display a snackbar
- Implement actions & shortcuts
- Manage keyboard focus
- Add assets and images
- Display images from the internet
- Fade in images with a placeholder
- Play and pause a video
- Add tabs to your app
- Navigate to a new screen and back
- Send data to a new screen
- Return data from a screen
- Add a drawer to a screen
- Setup deep linking
- Setup app links for Android
- Setup universal links for iOS
- Configure web URL strategies
- Implicit animations
- Animate the properties of a container
- Fade a widget in and out
- Hero animations
- Animate a page route transition
- Animate using a physic simulation
- Staggered animations
- Create a staggered menu animation
- API overview
- Accessibility
- Internationalization
- Think declaratively
- Ephemeral vs app state
- Simple app state management
- Fetch data from the internet
- Make authenticated requests
- Send data to the internet
- Update data over the internet
- Delete data on the internet
- Communicate with WebSockets
- JSON serialization
- Parse JSON in the background
- Store key-value data on disk
- Read and write files
- Persist data with SQLite
- Google APIs
- Supported platforms
- Build desktop apps with Flutter
- Write platform-specific code
- Automatic platform adaptations
- Add Android as build target
- Add a splash screen
- Bind to native code
- Host a native Android view
- Restore state on Android
- Target ChromeOS with Android
- Add iOS as build target
- Leverage Apple's system libraries
- Add a launch screen
- Add iOS App Clip support
- Add iOS app extensions
- Host a native iOS view
- Enable debugging on iOS
- Restore state on iOS
- Add Linux as build target
- Build a Linux app
- Add macOS as build target
- Build a macOS app
- Add web as build target
- Build a web app
- Web renderers
- Custom app initialization
- Display images on the web
- Add Windows as build target
- Build a Windows app
- Use packages & plugins
- Develop packages & plugins
- Flutter Favorites
- Package repository
- Testing overview
- Mock dependencies
- Find widgets
- Simulate scrolling
- Simulate user interaction
- Write and run an integration test
- Profile an integration test
- Test a plugin
- Handle plugin code in tests
- Debugging tools
- Debug your app programmatically
- Use a native language debugger
- Flutter's build modes
- Common Flutter errors
- Handle errors
- Report errors to a service
- Performance best practices
- Deferred components
- Rendering performance
- Performance profiling
- Performance profiling for web
- Shader compilation jank
- Performance metrics
- Concurrency and isolates
- Performance FAQ
- Obfuscate Dart code
- Create flavors of an app
- Build and release an Android app
- Build and release an iOS app
- Build and release a macOS app
- Build and release a Linux app
- Build and release a Windows app
- Build and release a web app
- Set up continuous deployment
- Set up Android project
- Add a single Flutter screen
- Add a Flutter Fragment
- Add a Flutter View
- Use a Flutter plugin
- Set up iOS project
- Debug embedded Flutter module
- Add multiple Flutter instances
- Loading sequence and performance
- Android Studio & IntelliJ
- Visual Studio Code
- Install from Android Studio & IntelliJ
- Install from VS Code
- Install from command line
- Flutter inspector
- Performance view
- CPU Profiler view
- Memory view
- Debug console view
- Network view
- Logging view
- App size tool
- DevTools extensions
- SDK overview
- Flutter's pubspec options
- Automated fixes
- Code formatting
- Architectural overview
- Inside Flutter
- Understanding constraints
- Contributing
- Create useful bug reports
- Contribute to Flutter
- Design documents
- Who is Dash?
- Widget index
- API reference
- flutter CLI reference

Web support for Flutter
Flutter’s web support delivers the same experiences on the web as on mobile. Building on the portability of Dart, the power of the web platform and the flexibility of the Flutter framework, you can now build apps for iOS, Android, and the browser from the same codebase. You can compile existing Flutter code written in Dart into a web experience because it is exactly the same Flutter framework and web is just another device target for your app.
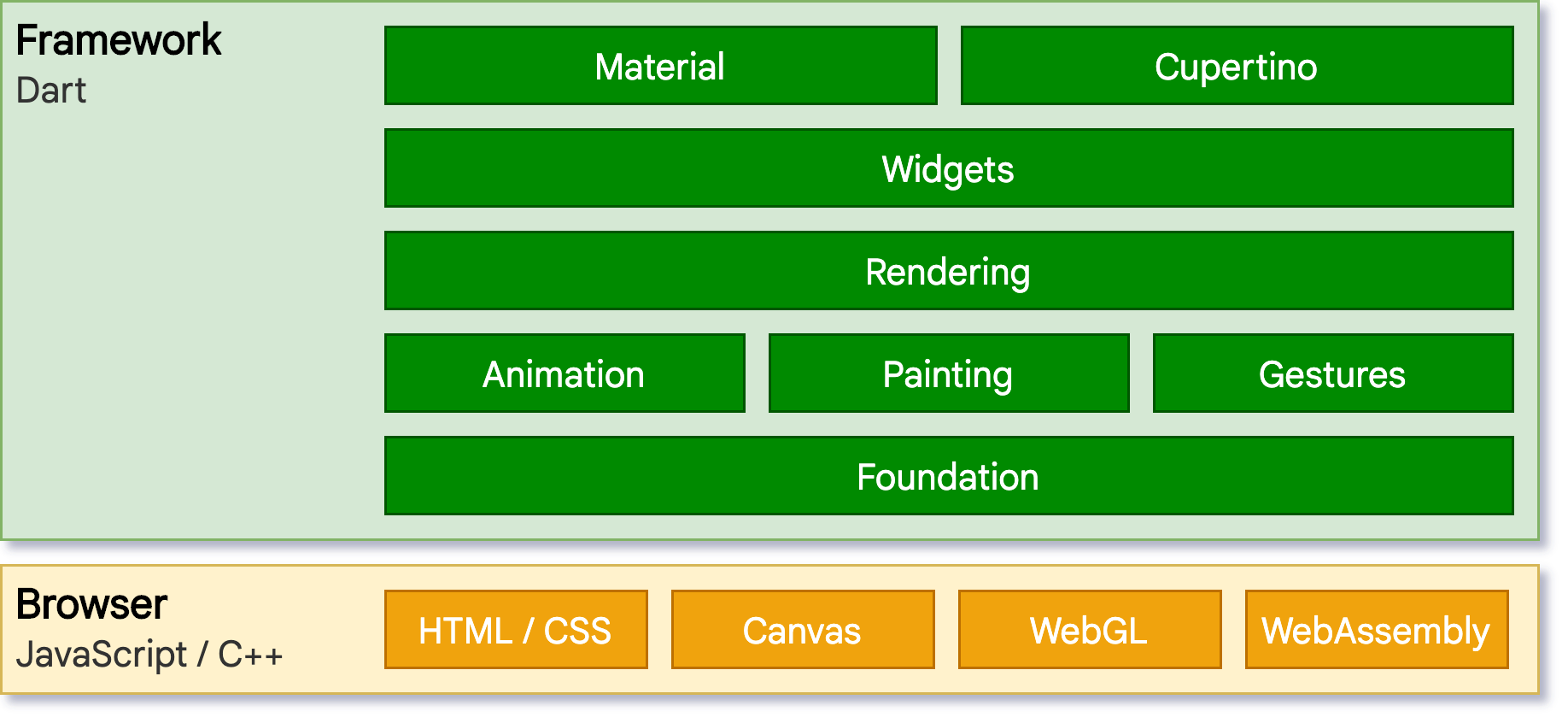
Adding web support to Flutter involved implementing Flutter’s core drawing layer on top of standard browser APIs, in addition to compiling Dart to JavaScript, instead of the ARM machine code that is used for mobile applications. Using a combination of DOM, Canvas, and WebAssembly, Flutter can provide a portable, high-quality, and performant user experience across modern browsers. We implemented the core drawing layer completely in Dart and used Dart’s optimized JavaScript compiler to compile the Flutter core and framework along with your application into a single, minified source file that can be deployed to any web server.
While you can do a lot on the web, Flutter’s web support is most valuable in the following scenarios:
Not every HTML scenario is ideally suited for Flutter at this time. For example, text-rich, flow-based, static content such as blog articles benefit from the document-centric model that the web is built around, rather than the app-centric services that a UI framework like Flutter can deliver. However, you can use Flutter to embed interactive experiences into these websites.
For a glimpse into how to migrate your mobile app to web, see the following video:
The following resources can help you get started:
- To add web support to an existing app, or to create a new app that includes web support, see Building a web application with Flutter .
- To learn about Flutter’s different web renderers (HTML and CanvasKit), see Web renderers
- To learn how to create a responsive Flutter app, see Creating responsive apps .
- To view commonly asked questions and answers, see the web FAQ .
- To see code examples, check out the web samples for Flutter .
- To see a Flutter web app demo, check out the Wonderous app .
- To learn about deploying a web app, see Preparing an app for web release .
- File an issue on the main Flutter repo.
- You can chat and ask web-related questions on the #help channel on Discord .

IMAGES
VIDEO
COMMENTS
To add web support to an existing project created using a previous version of Flutter, run the following command from your project’s top-level directory: content_copy. $ flutter create --platforms web . If you receive a not supported error, run the following command: content_copy. $ flutter config --enable-web.
To see code examples, check out the web samples for Flutter. To see a Flutter web app demo, check out the Wonderous app. To learn about deploying a web app, see Preparing an app for web release. File an issue on the main Flutter repo. You can chat and ask web-related questions on the #help channel on Discord.