JS Reference
Html events, html objects, other references, window history.back().
Create a back button on a page:
The output of the code above will be:
Click on Go Back to see how it works. (Will only work if a previous page exists in your history list)

Description
The history.back() method loads the previous URL (page) in the history list.
The history.back() method only works if a previous page exists.
history.back() is the same as history.go(-1) .
history.back() is the same as clicking "Back" your browser.
The history.forward() Method
The history.go() Method
The history.length Property
Return Value
Browser support.
history.back() is supported in all browsers:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Skip to main content
- Select language
- Skip to search
Manipulating the browser history
Moving to a specific point in history, reading the current state.
The DOM window object provides access to the browser's history through the history object. It exposes useful methods and properties that let you move back and forth through the user's history, as well as -- starting with HTML5 -- manipulate the contents of the history stack.
Traveling through history
Moving backward and forward through the user's history is done using the back() , forward() , and go() methods.
Moving forward and backward
To move backward through history, just do:
This will act exactly like the user clicked on the Back button in their browser toolbar.
Similarly, you can move forward (as if the user clicked the Forward button), like this:
You can use the go() method to load a specific page from session history, identified by its relative position to the current page (with the current page being, of course, relative index 0).
To move back one page (the equivalent of calling back() ):
To move forward a page, just like calling forward() :
Similarly, you can move forward 2 pages by passing 2, and so forth.
You can determine the number of pages in the history stack by looking at the value of the length property:
Adding and modifying history entries
HTML5 introduced the history.pushState() and history.replaceState() methods, which allow you to add and modify history entries, respectively. These methods work in conjunction with the window.onpopstate event.
Using history.pushState() changes the referrer that gets used in the HTTP header for XMLHttpRequest objects created after you change the state. The referrer will be the URL of the document whose window is this at the time of creation of the XMLHttpRequest object.
Example of pushState() method
Suppose http://mozilla.org/foo.html executes the following JavaScript:
This will cause the URL bar to display http://mozilla.org/bar.html , but won't cause the browser to load bar.html or even check that bar.html exists.
Suppose now that the user now navigates to http://google.com , then clicks back. At this point, the URL bar will display http://mozilla.org/bar.html , and the page will get a popstate event whose state object contains a copy of stateObj . The page itself will look like foo.html , although the page might modify its contents during the popstate event.
If we click back again, the URL will change to http://mozilla.org/foo.html , and the document will get another popstate event, this time with a null state object. Here too, going back doesn't change the document's contents from what they were in the previous step, although the document might update its contents manually upon receiving the popstate event.
The pushState() method
pushState() takes three parameters: a state object, a title (which is currently ignored), and (optionally) a URL. Let's examine each of these three parameters in more detail:
state object — The state object is a JavaScript object which is associated with the new history entry created by pushState() . Whenever the user navigates to the new state, a popstate event is fired, and the state property of the event contains a copy of the history entry's state object.
The state object can be anything that can be serialized. Because Firefox saves state objects to the user's disk so they can be restored after the user restarts the browser, we impose a size limit of 640k characters on the serialized representation of a state object. If you pass a state object whose serialized representation is larger than this to pushState() , the method will throw an exception. If you need more space than this, you're encouraged to use sessionStorage and/or localStorage .
title — Firefox currently ignores this parameter, although it may use it in the future. Passing the empty string here should be safe against future changes to the method. Alternatively, you could pass a short title for the state to which you're moving.
URL — The new history entry's URL is given by this parameter. Note that the browser won't attempt to load this URL after a call to pushState() , but it might attempt to load the URL later, for instance after the user restarts the browser. The new URL does not need to be absolute; if it's relative, it's resolved relative to the current URL. The new URL must be of the same origin as the current URL; otherwise, pushState() will throw an exception. This parameter is optional; if it isn't specified, it's set to the document's current URL.
In a sense, calling pushState() is similar to setting window.location = "#foo" , in that both will also create and activate another history entry associated with the current document. But pushState() has a few advantages:
- The new URL can be any URL in the same origin as the current URL. In contrast, setting window.location keeps you at the same document only if you modify only the hash.
- You don't have to change the URL if you don't want to. In contrast, setting window.location = "#foo"; only creates a new history entry if the current hash isn't #foo .
- You can associate arbitrary data with your new history entry. With the hash-based approach, you need to encode all of the relevant data into a short string.
- If title is subsequently used by browsers, this data can be utilized (independent of, say, the hash).
Note that pushState() never causes a hashchange event to be fired, even if the new URL differs from the old URL only in its hash.
In a XUL document, it creates the specified XUL element.
In other documents, it creates an element with a null namespace URI.
The replaceState() method
history.replaceState() operates exactly like history.pushState() except that replaceState() modifies the current history entry instead of creating a new one. Note that this doesn't prevent the creation of a new entry in the global browser history.
replaceState() is particularly useful when you want to update the state object or URL of the current history entry in response to some user action.
Example of replaceState() method
The explanation of these two lines above can be found at "Example of pushState() method" section. Then suppose http://mozilla.org/bar.html executes the following JavaScript:
This will cause the URL bar to display http://mozilla.org/bar2.html , but won't cause the browser to load bar2.html or even check that bar2.html exists.
Suppose now that the user now navigates to http://www.microsoft.com , then clicks back. At this point, the URL bar will display http://mozilla.org/bar2.html. If the user now clicks back again, the URL bar will display http://mozilla.org/foo.html, and totaly bypass bar.html.
The popstate event
A popstate event is dispatched to the window every time the active history entry changes. If the history entry being activated was created by a call to pushState or affected by a call to replaceState , the popstate event's state property contains a copy of the history entry's state object.
See window.onpopstate for sample usage.
When your page loads, it might have a non-null state object. This can happen, for example, if the page sets a state object (using pushState() or replaceState() ) and then the user restarts their browser. When your page reloads, the page will receive an onload event, but no popstate event. However, if you read the history.state property, you'll get back the state object you would have gotten if a popstate had fired.
You can read the state of the current history entry without waiting for a popstate event using the history.state property like this:
For a complete example of AJAX web site, please see: Ajax navigation example .
Specifications
Browser compatibility.
- window.history
- window.onpopstate
Document Tags and Contributors
- Skip to main content
- Select language
- Skip to search
- Sign in Github
Specifications
Browser compatibility.
The Window . history read-only property returns a reference to the History object, which provides an interface for manipulating the browser session history (pages visited in the tab or frame that the current page is loaded in).
See Manipulating the browser history for examples and details. In particular, that article explains security features of the pushState() and replaceState() methods that you should be aware of before using them.
For top-level pages you can see the list of pages in the session history, accessible via the History object, in the browser's dropdowns next to the back and forward buttons.
For security reasons the History object doesn't allow the non-privileged code to access the URLs of other pages in the session history, but it does allow it to navigate the session history.
There is no way to clear the session history or to disable the back/forward navigation from unprivileged code. The closest available solution is the location.replace() method, which replaces the current item of the session history with the provided URL.
Document Tags and Contributors
- History API
- NeedsExample
- NeedsMarkupWork
- applicationCache
- controllers
- customElements
- defaultStatus
- devicePixelRatio
- dialogArguments
- directories
- or ) in which the window is embedded, or null if the element is either top-level or is embedded into a document with a different script origin; that is, in cross-origin situations."> frameElement
- innerHeight
- isSecureContext
- or elements) in the window."> length
- localStorage
- locationbar
- mozAnimationStartTime
- mozInnerScreenX
- mozInnerScreenY
- mozPaintCount
- onafterprint
- onanimationcancel
- onanimationend
- onanimationiteration
- onappinstalled
- onbeforeinstallprompt
- onbeforeprint
- onbeforeunload
- element."> oncancel
- oncanplaythrough
- element."> onclose
- oncontextmenu
- oncuechange
- ondevicelight
- ondevicemotion
- ondeviceorientation
- ondeviceorientationabsolute
- ondeviceproximity
- ondurationchange
- ongamepadconnected
- ongamepaddisconnected
- ongotpointercapture
- onhashchange
- , , and elements. It also handles these events on elements where contenteditable or designMode are turned on."> oninput
- onlanguagechange
- element, etc."> onload
- onloadeddata
- onloadedmetadata
- onloadstart
- onlostpointercapture
- onmessageerror
- onmousedown
- onmouseenter
- onmouseleave
- onmousemove
- onmouseover
- onmozbeforepaint
- onpointercancel
- onpointerdown
- onpointerenter
- onpointerleave
- onpointermove
- onpointerout
- onpointerover
- onpointerup
- onrejectionhandled
- onselectionchange
- onselectstart
- ontouchcancel
- ontouchstart
- ontransitioncancel
- ontransitionend
- onunhandledrejection
- onuserproximity
- onvrdisplayactivate
- onvrdisplayblur
- onvrdisplayconnect
- onvrdisplaydeactivate
- onvrdisplaydisconnect
- onvrdisplayfocus
- onvrdisplaypointerrestricted
- onvrdisplaypointerunrestricted
- onvrdisplaypresentchange
- outerHeight
- pageXOffset
- pageYOffset
- performance
- personalbar
- sessionStorage
- speechSynthesis
- visualViewport
- windowState
- cancelAnimationFrame()
- cancelIdleCallback()
- captureEvents()
- clearImmediate()
- clearInterval()
- clearTimeout()
- convertPointFromNodeToPage()
- convertPointFromPageToNode
- createImageBitmap()
- getAttention()
- getComputedStyle()
- getDefaultComputedStyle()
- getSelection()
- matchMedia()
- or tab) with the specified name. If the name doesn't exist, then a new window is opened and the specified resource is loaded into its browsing context."> open()
- openDialog()
- postMessage()
- releaseEvents()
- requestAnimationFrame()
- requestFileSystem()
- requestIdleCallback()
- routeEvent()
- scrollByLines()
- scrollByPages()
- setCursor()
- setImmediate()
- setInterval()
- setTimeout()
- showModalDialog()
- sizeToContent()
- updateCommands()
- beforeprint
- beforeunload
- DOMContentLoaded
- languagechange
- messageerror
- orientationchange
- rejectionhandled
- unhandledrejection
- vrdisplayconnect
- vrdisplaydisconnect
- vrdisplaypresentchange
Learn the best of web development
Get the latest and greatest from MDN delivered straight to your inbox.
Thanks! Please check your inbox to confirm your subscription.
If you haven’t previously confirmed a subscription to a Mozilla-related newsletter you may have to do so. Please check your inbox or your spam filter for an email from us.
- The Best Tech Gifts Under $100
- Traveling? Get These Gadgets!
How to Reopen Closed Safari Tabs and Windows and Access Past History
Quickly get back to where you were
Tom Nelson is an engineer, programmer, network manager, and computer network and systems designer who has written for Other World Computing,and others. Tom is also president of Coyote Moon, Inc., a Macintosh and Windows consulting firm.
What to Know
- Undo a closed tab: Go to Edit > Undo Close Tab , press Command + Z , or click and hold the plus sign to the right of the Tabs bar.
- Or, select History > Reopen Last Closed Tab , go to History and mouse over Recently Closed , or press Shift + Command + T .
- Restore a closed window: Go to History > Reopen Last Closed Window . Or, History > Reopen All Windows From Last Session .
This article explains how to reopen tabs or windows you may have closed by accident in the Safari web browser. You can also use the History list to reopen sites.
How to Undo a Closed Tab in Safari
You can open your lost tab using four methods. The first is to either select Undo Close Tab from the Edit menu, or press Command + Z on your keyboard.
You can restore multiple tabs you've closed by using the command repeatedly.
Another way to reopen a page you've closed is to click and hold the plus sign at the far right of the Tabs bar. Usually, you click this once to open a new tab, but holding opens a menu with a list of ones you've recently closed. Select the one you want to reopen.
The third way is to select Reopen Last Closed Tab under the History menu or press Shift + Command + T on your keyboard.
Finally, you can find a list of tabs you've recently closed under the History menu. Mouse over Recently Closed to see a list of pages you can reopen, and then click the one you want to restore.
Restoring Closed Windows
If you close a Safari window , you can reopen it just as you can reopen a closed tab, but the command is under a different menu. Select Reopen Last Closed Window under the History menu, or press Shift + Command + T on your keyboard.
The Reopen Last Closed Window and Reopen Last Closed Tab commands share the same spot in the History menu and keyboard shortcut. Which you see depends on which you closed most recently.
Reopen Safari Windows From Last Session
Besides being able to reopen closed Safari windows and tabs, you can also open all Safari windows that were open the last time you quit Safari.
Safari, like all the Apple apps, can make use of OS X’s Resume feature , which was introduced with OS X Lion. Resume saves the state of all the open windows of an app, in this case, any Safari window you have open. The idea is that the next time you launch Safari, you can resume right where you left off.
From the History menu, select Reopen All Windows From Last Session .
Get the Latest Tech News Delivered Every Day
- How to Restore Tabs in Chrome
- How to Force-Quit a Program (Windows 10, 8, 7, Vista, XP)
- How to Undo and Redo on a Mac
- The Best Windows Keyboard Shortcuts in 2024
- Using Finder Tabs in OS X
- How to Use Group Tabs in Chrome
- The Best Mac Shortcuts in 2024
- How to Use Sticky Notes for Mac
- The 20 Best Firefox Extensions of 2024
- Customize Safari Toolbar, Favorites, Tab, and Status Bars
- How to Bookmark All Tabs in Chrome on Android
- Keyboard Shortcuts for Safari on macOS
- 8 Tips for Using Safari With macOS
- How to Pin Sites in Safari and Mac OS
- Control Safari Windows With Keyboard Shortcuts
- How to Bring Back the Old Context Menu in Windows 11
Manipulating the browser history
The DOM window object provides access to the browser's history through the history object. It exposes useful methods and properties that let you move back and forth through the user's history, as well as -- starting with HTML5 -- manipulate the contents of the history stack.
Traveling through history
Moving backward and forward through the user's history is done using the back() , forward() , and go() methods.
Moving forward and backward
To move backward through history, just do:
This will act exactly like the user clicked on the Back button in their browser toolbar.
Similarly, you can move forward (as if the user clicked the Forward button), like this:
Moving to a specific point in history
You can use the go() method to load a specific page from session history, identified by its relative position to the current page (with the current page being, of course, relative index 0).
To move back one page (the equivalent of calling back() ):
To move forward a page, just like calling forward() :
Similarly, you can move forward 2 pages by passing 2, and so forth.
You can determine the number of pages in the history stack by looking at the value of the length property:
Adding and modifying history entries
HTML5 introduced the history.pushState() and history.replaceState() methods, which allow you to add and modify history entries, respectively. These methods work in conjunction with the window.onpopstate event.
Using history.pushState() changes the referrer that gets used in the HTTP header for XMLHttpRequest objects created after you change the state. The referrer will be the URL of the document whose window is this at the time of creation of the XMLHttpRequest object.
Suppose http://mozilla.org/foo.html executes the following JavaScript:
This will cause the URL bar to display http://mozilla.org/bar.html , but won't cause the browser to load bar.html or even check that bar.html exists.
Suppose now that the user now navigates to http://google.com , then clicks back. At this point, the URL bar will display http://mozilla.org/bar.html , and the page will get a popstate event whose state object contains a copy of stateObj . The page itself will look like foo.html , although the page might modify its contents during the popstate event.
If we click back again, the URL will change to http://mozilla.org/foo.html , and the document will get another popstate event, this time with a null state object. Here too, going back doesn't change the document's contents from what they were in the previous step, although the document might update its contents manually upon receiving the popstate event.
The pushState() method
pushState() takes three parameters: a state object, a title (which is currently ignored), and (optionally) a URL. Let's examine each of these three parameters in more detail:
state object — The state object is a JavaScript object which is associated with the new history entry created by pushState() . Whenever the user navigates to the new state, a popstate event is fired, and the state property of the event contains a copy of the history entry's state object.
The state object can be anything that can be serialized. Because Firefox saves state objects to the user's disk so they can be restored after the user restarts the browser, we impose a size limit of 640k characters on the serialized representation of a state object. If you pass a state object whose serialized representation is larger than this to pushState() , the method will throw an exception. If you need more space than this, you're encouraged to use sessionStorage and/or localStorage .
title — Firefox currently ignores this parameter, although it may use it in the future. Passing the empty string here should be safe against future changes to the method. Alternatively, you could pass a short title for the state to which you're moving.
URL — The new history entry's URL is given by this parameter. Note that the browser won't attempt to load this URL after a call to pushState() , but it might attempt to load the URL later, for instance after the user restarts the browser. The new URL does not need to be absolute; if it's relative, it's resolved relative to the current URL. The new URL must be of the same origin as the current URL; otherwise, pushState() will throw an exception. This parameter is optional; if it isn't specified, it's set to the document's current URL.
In a sense, calling pushState() is similar to setting window.location = "#foo" , in that both will also create and activate another history entry associated with the current document. But pushState() has a few advantages:
- The new URL can be any URL in the same origin as the current URL. In contrast, setting window.location keeps you at the same document only if you modify only the hash.
- You don't have to change the URL if you don't want to. In contrast, setting window.location = "#foo"; only creates a new history entry if the current hash isn't #foo .
- You can associate arbitrary data with your new history entry. With the hash-based approach, you need to encode all of the relevant data into a short string.
- If title is subsequently used by browsers, this data can be utilized (independent of, say, the hash).
Note that pushState() never causes a hashchange event to be fired, even if the new URL differs from the old URL only in its hash.
In a XUL document, it creates the specified XUL element.
In other documents, it creates an element with a null namespace URI.
The replaceState() method
history.replaceState() operates exactly like history.pushState() except that replaceState() modifies the current history entry instead of creating a new one. Note that this doesn't prevent the creation of a new entry in the global browser history.
replaceState() is particularly useful when you want to update the state object or URL of the current history entry in response to some user action.
The popstate event
A popstate event is dispatched to the window every time the active history entry changes. If the history entry being activated was created by a call to pushState or affected by a call to replaceState , the popstate event's state property contains a copy of the history entry's state object.
See window.onpopstate for sample usage.
Reading the current state
When your page loads, it might have a non-null state object. This can happen, for example, if the page sets a state object (using pushState() or replaceState() ) and then the user restarts their browser. When your page reloads, the page will receive an onload event, but no popstate event. However, if you read the history.state property, you'll get back the state object you would have gotten if a popstate had fired.
You can read the state of the current history entry without waiting for a popstate event using the history.state property like this:
For a complete example of AJAX web site, please see: Ajax navigation example .
Specifications
Browser compatibility.
- window.history
- window.onpopstate
© 2016 Mozilla Contributors Licensed under the Creative Commons Attribution-ShareAlike License v2.5 or later. https://developer.mozilla.org/en-us/docs/web/api/history_api
- PRO Courses Guides New Tech Help Pro Expert Videos About wikiHow Pro Upgrade Sign In
- EDIT Edit this Article
- EXPLORE Tech Help Pro About Us Random Article Quizzes Request a New Article Community Dashboard This Or That Game Popular Categories Arts and Entertainment Artwork Books Movies Computers and Electronics Computers Phone Skills Technology Hacks Health Men's Health Mental Health Women's Health Relationships Dating Love Relationship Issues Hobbies and Crafts Crafts Drawing Games Education & Communication Communication Skills Personal Development Studying Personal Care and Style Fashion Hair Care Personal Hygiene Youth Personal Care School Stuff Dating All Categories Arts and Entertainment Finance and Business Home and Garden Relationship Quizzes Cars & Other Vehicles Food and Entertaining Personal Care and Style Sports and Fitness Computers and Electronics Health Pets and Animals Travel Education & Communication Hobbies and Crafts Philosophy and Religion Work World Family Life Holidays and Traditions Relationships Youth
- Browse Articles
- Learn Something New
- Quizzes Hot
- This Or That Game New
- Train Your Brain
- Explore More
- Support wikiHow
- About wikiHow
- Log in / Sign up
- Computers and Electronics
- Internet Browsers
- Safari Browser
2 Easy Ways to Check Your Safari Search History
Last Updated: January 17, 2024 Fact Checked
This article was co-authored by wikiHow staff writer, Rain Kengly . Rain Kengly is a wikiHow Technology Writer. As a storytelling enthusiast with a penchant for technology, they hope to create long-lasting connections with readers from all around the globe. Rain graduated from San Francisco State University with a BA in Cinema. This article has been fact-checked, ensuring the accuracy of any cited facts and confirming the authority of its sources. This article has been viewed 156,346 times. Learn more...
Do you need to check your Safari browser history? In a few simple steps, you can easily view all the websites you accessed in the past. You can also search for specific websites in your history and clear any website data you don't want. This wikiHow will teach you how to view and delete your Safari history on iPhone, iPad, and macOS.
Things You Should Know
- On iPhone and iPad, you can click the book icon, then the clock icon to find your Safari history.
- On macOS, you can click the "History" tab, then "Show History".
- You can search for specific websites and clear your history data.
Using iPhone and iPad

- If needed, you can change your General Settings .

- If you're signed in with the same Apple ID to you use to log into your Mac, your Mac's Safari history will appear in this list as well.

- You may have to swipe down on the History page to find it.
- The results will load as you type.

- Tap a time period to delete the history from just that time period. To delete the entire log, select All time .
- You can also swipe left on individual websites in your history, then click Delete .
Using macOS

- Be sure to update Safari.

- If you're logged into the computer with the same Apple ID you use on your iPhone or iPad, you'll also see sites you've visited on those devices.

- A list of matching results from your history will appear. Click a site to load it in Safari.

- Select a time frame from the drop-down menu, then click Clear History .
- You can also delete cookies from Safari .
Expert Q&A
You Might Also Like

- ↑ https://support.apple.com/guide/safari/search-your-browsing-history-ibrw1114/mac
- ↑ https://support.apple.com/en-us/HT201265
About This Article

iPhone/iPad: 1. Open Safari . 2. Tap the book icon. 3. Tap the clock button. macOS: 1. Open Safari . 2. Click History . 3. Click Show All History . Did this summary help you? Yes No
- Send fan mail to authors
Is this article up to date?


Featured Articles

Trending Articles

Watch Articles

- Terms of Use
- Privacy Policy
- Do Not Sell or Share My Info
- Not Selling Info
wikiHow Tech Help Pro:
Level up your tech skills and stay ahead of the curve
Clear the history, cache, and cookies from Safari on your iPhone, iPad, or iPod touch
Learn how to delete your history, cookies, and cache in Settings.
Delete history, cache, and cookies
Clear cookies and cache, but keep your history, delete a website from your history, block cookies, use content blockers.
Go to Settings > Safari.
Tap Clear History and Website Data.
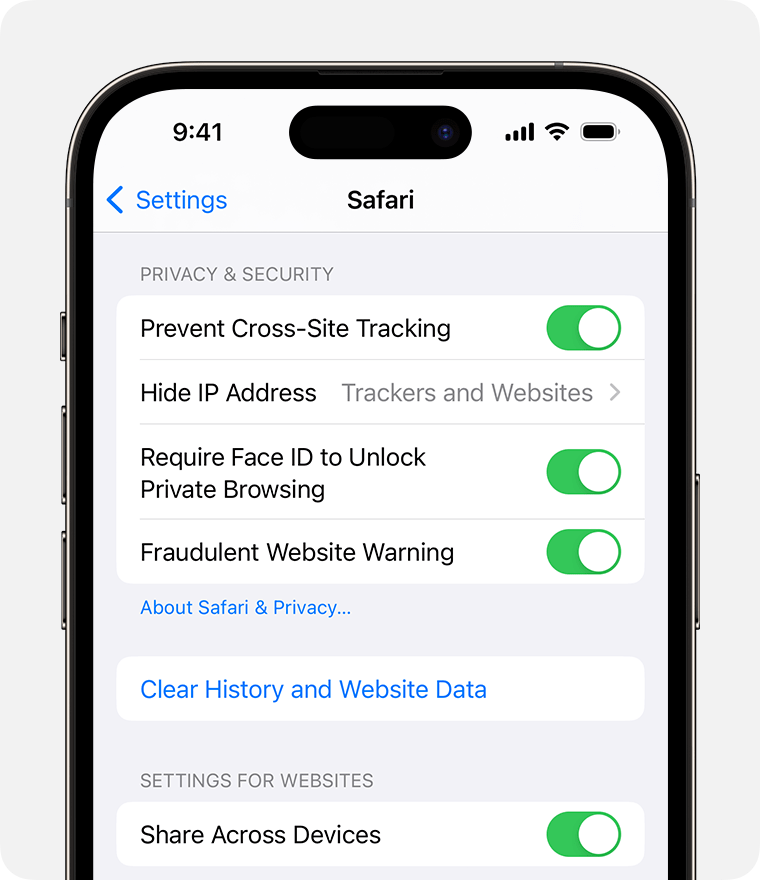
Clearing your history, cookies, and browsing data from Safari won't change your AutoFill information.
When there's no history or website data to clear, the button to clear it turns gray. The button might also be gray if you have web content restrictions set up under Content & Privacy Restrictions in Screen Time .
To visit sites without leaving a history, turn Private Browsing on .
Go to Settings > Safari > Advanced > Website Data.
Tap Remove All Website Data.
When there's no website data to clear, the button to clear it turns gray. The button might also be gray if you have web content restrictions set up under Content & Privacy Restrictions in Screen Time .
Open the Safari app.

Tap the Edit button, then select the website or websites that you want to delete from your history.
Tap the Delete button.
A cookie is a piece of data that a site puts on your device so that site can remember you when you visit again.
To block cookies:
Go to Settings > Safari > Advanced.
Turn on Block All Cookies.
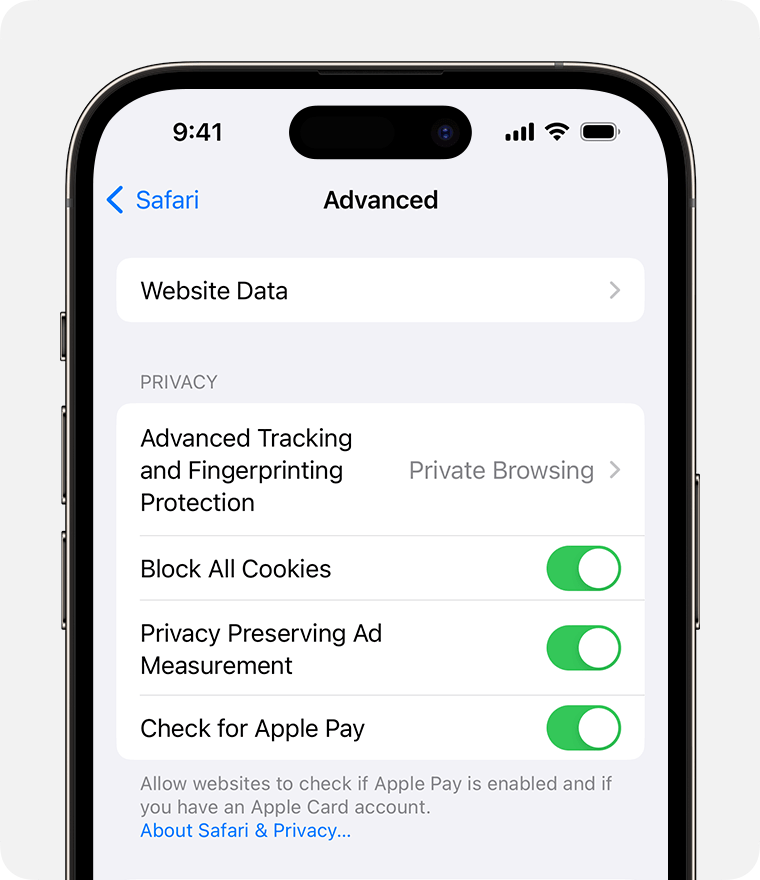
If you block cookies, some web pages might not work. Here are some examples:
You will likely not be able to sign in to a site even when using your correct username and password.
You might see a message that cookies are required or that your browser's cookies are off.
Some features on a site might not work.
Content blockers are third-party apps and extensions that let Safari block cookies, images, resources, pop-ups, and other content.
To get a content blocker:
Download a content blocking app from the App Store.
Tap Settings > Safari > Extensions.
Tap to turn on a listed content blocker.
You can use more than one content blocker. If you need help, contact the app developer .
Information about products not manufactured by Apple, or independent websites not controlled or tested by Apple, is provided without recommendation or endorsement. Apple assumes no responsibility with regard to the selection, performance, or use of third-party websites or products. Apple makes no representations regarding third-party website accuracy or reliability. Contact the vendor for additional information.
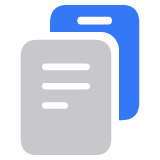
Related topics
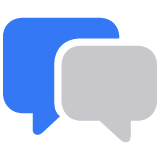
Explore Apple Support Community
Find what’s been asked and answered by Apple customers.
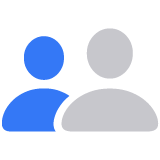
Contact Apple Support
Need more help? Save time by starting your support request online and we'll connect you to an expert.
Demystifying JavaScript history.back() and history.go() methods
Ciprian on Tuesday, May 17, 2022 in AJAX and Fetching Data Last modified on Friday, June 3, 2022
One of my clients needed his online shop customers to be able to go back several steps with one click and keep the current session variables in memory. The obvious solution was the browser’s back button. But I couldn’t instruct the customer to click it one time or 3 times or 5 times. So, I chose to add a link with a JavaScript history object.
back() – Go to the previous URL entry in the history list. This does the same thing as the browser’s back button.
forward() – Go to the next URL entry in the history list. This does the same thing as the browser’s forward button. This is only effective when there is a next document in the history list. The back function or browser’s back button must have previously been used for this function to work.
go(position) – This function will accept an integer or a string. If an integer is used, the browser will go forward or back (if the value is negative) the number of specified pages in the history object (if the requested entry exists).
<a href="javascript:history.back()">Go back</a>
<a href="javascript:history.go(-3)">Go back to step X</a>
The back() and go() methods are supported in all major browsers.
History is one of the sub-objects of Windows, and an interface to the history stored by your browser. Properties and methods apply to the object history of windows or to each frame in the window.
history.go(0) acts like pressing the reload button.
The length property returns the number of URLs in the history list.
Note: Internet Explorer and Opera start at 0, while Firefox, Chrome, and Safari start at 1.
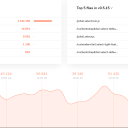
tail.select takeover and update
How to get content from another website using javascript.
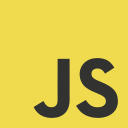
Related posts
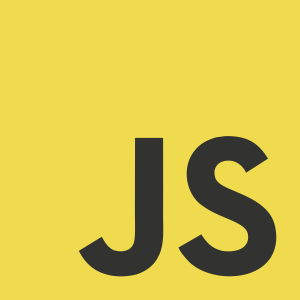
April 23, 2023
Check DNS records in bulk using JavaScript
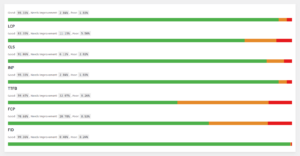
May 30, 2023
How to set up your own Google CrUX report
May 27, 2022
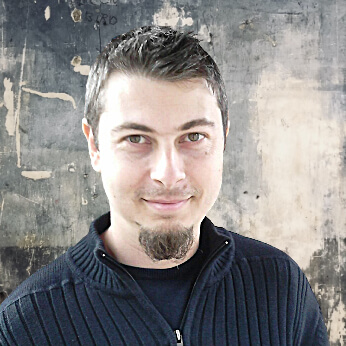
Ciprian Popescu
Technical SEO specialist , JavaScript developer , WordPress developer , and senior full-stack developer.
Latest Articles
What’s new in the latest lighthouse release.
Monday, April 22, 2024
Dark Mode for Lighthouse!
Friday, March 8, 2024
WordPress Plugins and User Experience
Friday, March 1, 2024
Unlimited Licenses Are Back!
Monday, February 26, 2024
How to Build a Front-end Password Reset Form in WordPress
Friday, February 23, 2024
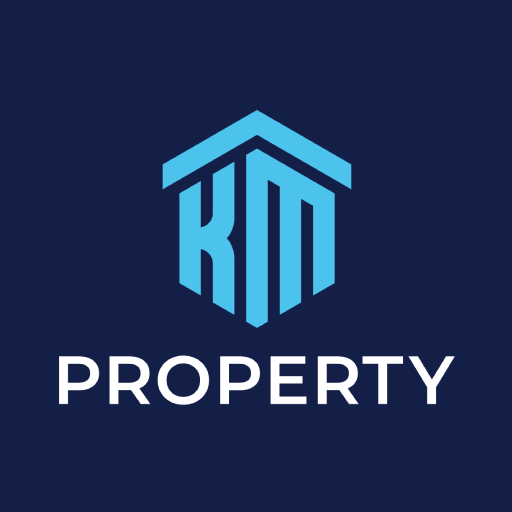
getButterfly is a personal web development blog in Dublin, Ireland.
Privacy Policy Terms of Service Support JavaScript Legacy About getButterfly.com Knowledge Base Bots Buy me a coffee @CodeCanyon @WordPress @linktr.ee Status
Image Compressor NEW — Compress and optimize your PNG/JPG images.
Copyright ©2005-2024 getButterfly. All Rights Reserved. v 6.7.12
How To Get Safari Back To Normal

- Software & Applications
- Browsers & Extensions
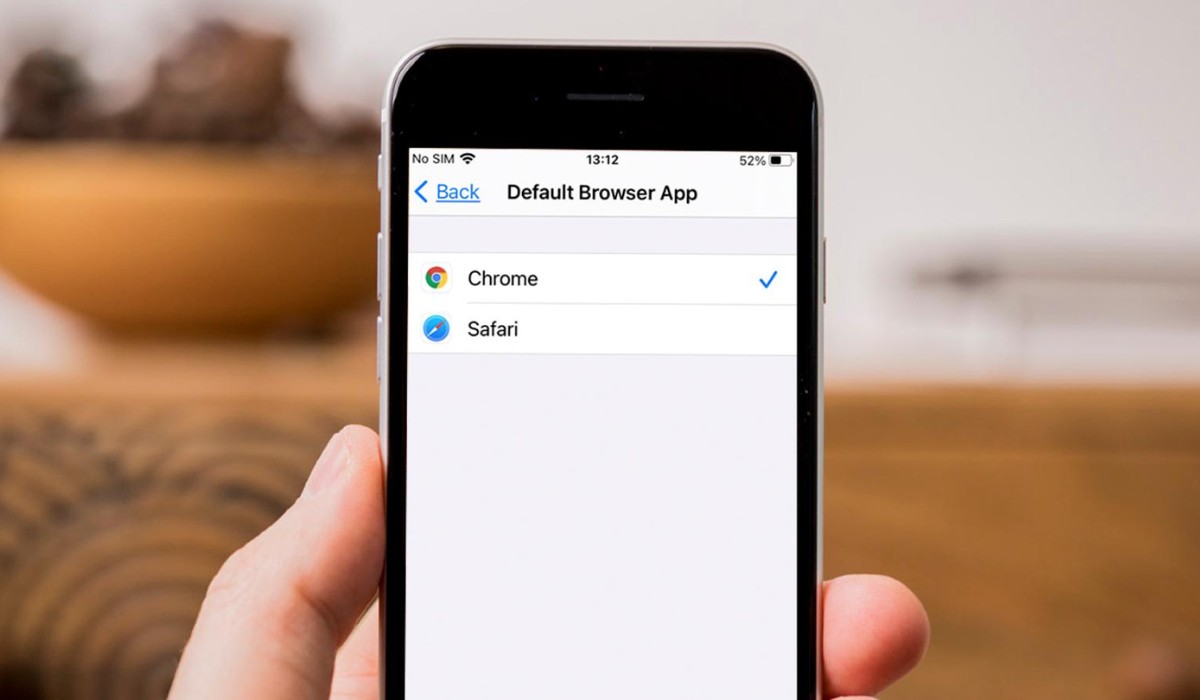
Clear Safari Cache and History
Clearing the cache and history in Safari can help resolve various issues, such as slow performance, unresponsive web pages, and excessive memory usage. It's a simple yet effective way to refresh the browser and get it back to its optimal state. Here's how you can clear the cache and history in Safari:
Open Safari Preferences : Launch Safari and click on "Safari" in the top menu bar. From the drop-down menu, select "Preferences."
Navigate to the Privacy Tab : In the Preferences window, click on the "Privacy" tab. This is where you can manage your browsing data.
Manage Website Data : Under the "Privacy" tab, you'll find the "Manage Website Data" button. Click on it to view the stored website data, including cache and cookies.
Remove All Website Data : To clear the cache and history, click on "Remove All Website Data." Safari will prompt you to confirm the action. Click "Remove Now" to proceed.
Clear History : After removing the website data, you can also clear your browsing history. In the Safari Preferences window, navigate to the "History" tab and click on "Clear History." You can choose the time range for which you want to clear the history and then click "Clear History."
Restart Safari : Once you've cleared the cache and history, it's a good idea to restart Safari to ensure that the changes take effect.
By following these steps, you can effectively clear the cache and history in Safari, giving the browser a fresh start and potentially resolving any performance issues you may have been experiencing.
Disable Safari Extensions
Safari extensions are add-ons that enhance the functionality of the browser by providing additional features and customization options. While these extensions can be incredibly useful, they can also sometimes cause issues with the browser's performance. If you're experiencing problems with Safari, such as slow loading times, frequent crashes, or unresponsive web pages, disabling extensions can be a helpful troubleshooting step.
Here's how you can disable Safari extensions:
Navigate to the Extensions Tab : In the Preferences window, click on the "Extensions" tab. This is where you can manage all the installed extensions in Safari.
Disable Extensions : You'll see a list of all the installed extensions along with checkboxes next to each one. To disable an extension, simply uncheck the box next to it. This will effectively disable the extension without uninstalling it.
Remove Extensions : If you want to completely remove an extension from Safari, you can click on the extension and select "Uninstall." This will remove the extension from Safari entirely.
Restart Safari : After disabling or removing extensions, it's a good idea to restart Safari to ensure that the changes take effect.
By disabling or removing extensions, you can troubleshoot issues related to Safari's performance and stability. If you notice an improvement in the browser's behavior after disabling certain extensions, you may consider re-enabling them one by one to identify the problematic extension. This process can help pinpoint the specific extension causing issues and allow you to take appropriate action, such as updating or removing the problematic extension.
Remember that while extensions can add valuable functionality to Safari, having too many active extensions or using outdated or incompatible ones can impact the browser's performance. Regularly reviewing and managing your extensions can help keep Safari running smoothly and ensure a seamless browsing experience.
Taking the time to disable or remove problematic extensions can contribute to restoring Safari to its normal state, allowing you to enjoy a more reliable and efficient browsing experience.
Reset Safari Settings
Resetting Safari settings can be a valuable troubleshooting step when you encounter persistent issues with the browser. It essentially reverts the browser to its default state, eliminating any customizations or configurations that may be contributing to the problems you're experiencing. Whether you're dealing with frequent crashes, unresponsive web pages, or erratic behavior, resetting Safari settings can help restore the browser to a stable and functional state.
Here's how you can reset Safari settings:
Navigate to the Advanced Tab : In the Preferences window, click on the "Advanced" tab. This is where you can access advanced settings and configurations for Safari.
Enable Develop Menu : At the bottom of the Preferences window, check the box next to "Show Develop menu in menu bar." This will enable the Develop menu in Safari.
Access Develop Menu : After enabling the Develop menu, you'll see it appear in the top menu bar. Click on "Develop" and select "Empty Caches." This will clear the cached data in Safari.
Reset Safari : Still within the Develop menu, you'll find the option to "Reset Safari." Click on this option to open the reset dialog box.
Select Reset Options : In the reset dialog box, you'll have the opportunity to choose which settings you want to reset. You can reset various aspects of Safari, including history, top sites, and more. Select the options that align with the issues you're facing.
Confirm the Reset : After selecting the reset options, click "Reset" to confirm the action. Safari will proceed to reset the chosen settings to their default state.
Restart Safari : Once the reset process is complete, it's recommended to restart Safari to ensure that the changes take effect.
By following these steps, you can effectively reset Safari settings, potentially resolving persistent issues and restoring the browser to a stable and functional state. It's important to note that resetting Safari settings will remove any customizations, saved passwords, and website data, so it's advisable to consider this option when other troubleshooting steps have been unsuccessful.
Resetting Safari settings can serve as a powerful tool for addressing various issues, providing a fresh start for the browser and allowing you to enjoy a smoother and more reliable browsing experience. Whether you're encountering performance issues, unexpected behavior, or other concerns, resetting Safari settings can help bring the browser back to normal, ensuring that you can navigate the web with confidence and efficiency.
Update Safari to the Latest Version
Keeping Safari up to date with the latest version is crucial for ensuring optimal performance, security, and compatibility with modern websites and web applications. Apple regularly releases updates for Safari, addressing vulnerabilities, introducing new features, and enhancing overall stability. By updating Safari to the latest version, you can benefit from improved browsing experiences and safeguard your online activities. Here's how you can update Safari to the latest version:
Check for Updates : Launch Safari and click on "Safari" in the top menu bar. From the drop-down menu, select "About Safari." A window will appear, indicating the current version of Safari installed on your system. Next to the version number, there will be an option to "Check for Updates." Click on this to initiate the update process.
Install Available Updates : If a new version of Safari is available, you will be prompted to install it. Follow the on-screen instructions to download and install the latest update. Depending on your system settings, you may need to enter your administrator password to authorize the installation.
Automatic Updates : To ensure that Safari stays up to date automatically, you can enable the "Install system data files and security updates" option in the "Software Update" section of System Preferences. This will allow Safari to receive updates along with other system components, keeping it current without requiring manual intervention.
Restart Safari : After updating Safari to the latest version, it's advisable to restart the browser to ensure that the changes take effect. This can help prevent any lingering issues related to the previous version and ensure that you're benefiting from the latest improvements and security enhancements.
Updating Safari to the latest version is a proactive measure that can enhance your browsing security, protect your personal information, and provide access to the latest web technologies. By staying current with Safari updates, you can enjoy a more secure and reliable browsing experience, benefiting from improved performance and compatibility with evolving web standards.
Regularly checking for and installing Safari updates is a simple yet effective way to ensure that your browser remains in peak condition, allowing you to explore the web with confidence and peace of mind. Whether you're using Safari for work, leisure, or both, keeping it up to date is essential for staying connected, productive, and secure in the digital landscape.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Crowdfunding
- Cryptocurrency
- Digital Banking
- Digital Payments
- Investments
- Console Gaming
- Mobile Gaming
- VR/AR Gaming
- Gadget Usage
- Gaming Tips
- Online Safety
- Software Tutorials
- Tech Setup & Troubleshooting
- Buyer’s Guides
- Comparative Analysis
- Gadget Reviews
- Service Reviews
- Software Reviews
- Mobile Devices
- PCs & Laptops
- Smart Home Gadgets
- Content Creation Tools
- Digital Photography
- Video & Music Streaming
- Online Security
- Online Services
- Web Hosting
- WiFi & Ethernet
- Browsers & Extensions
- Communication Platforms
- Operating Systems
- Productivity Tools
- AI & Machine Learning
- Cybersecurity
- Emerging Tech
- IoT & Smart Devices
- Virtual & Augmented Reality
- Latest News
- AI Developments
- Fintech Updates
- Gaming News
- New Product Launches

- Fintechs and Traditional Banks Navigating the Future of Financial Services
- AI Writing How Its Changing the Way We Create Content
Related Post
How to find the best midjourney alternative in 2024: a guide to ai anime generators, unleashing young geniuses: how lingokids makes learning a blast, 10 best ai math solvers for instant homework solutions, 10 best ai homework helper tools to get instant homework help, 10 best ai humanizers to humanize ai text with ease, sla network: benefits, advantages, satisfaction of both parties to the contract, related posts.
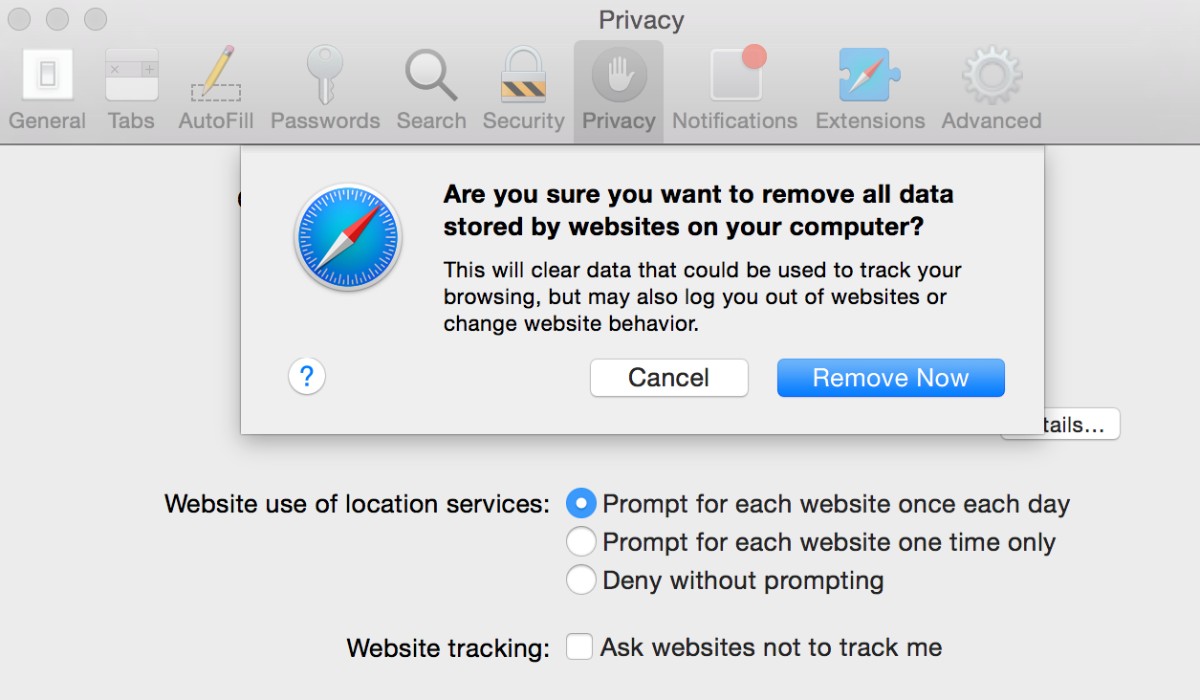
How To Restart Safari On Macbook
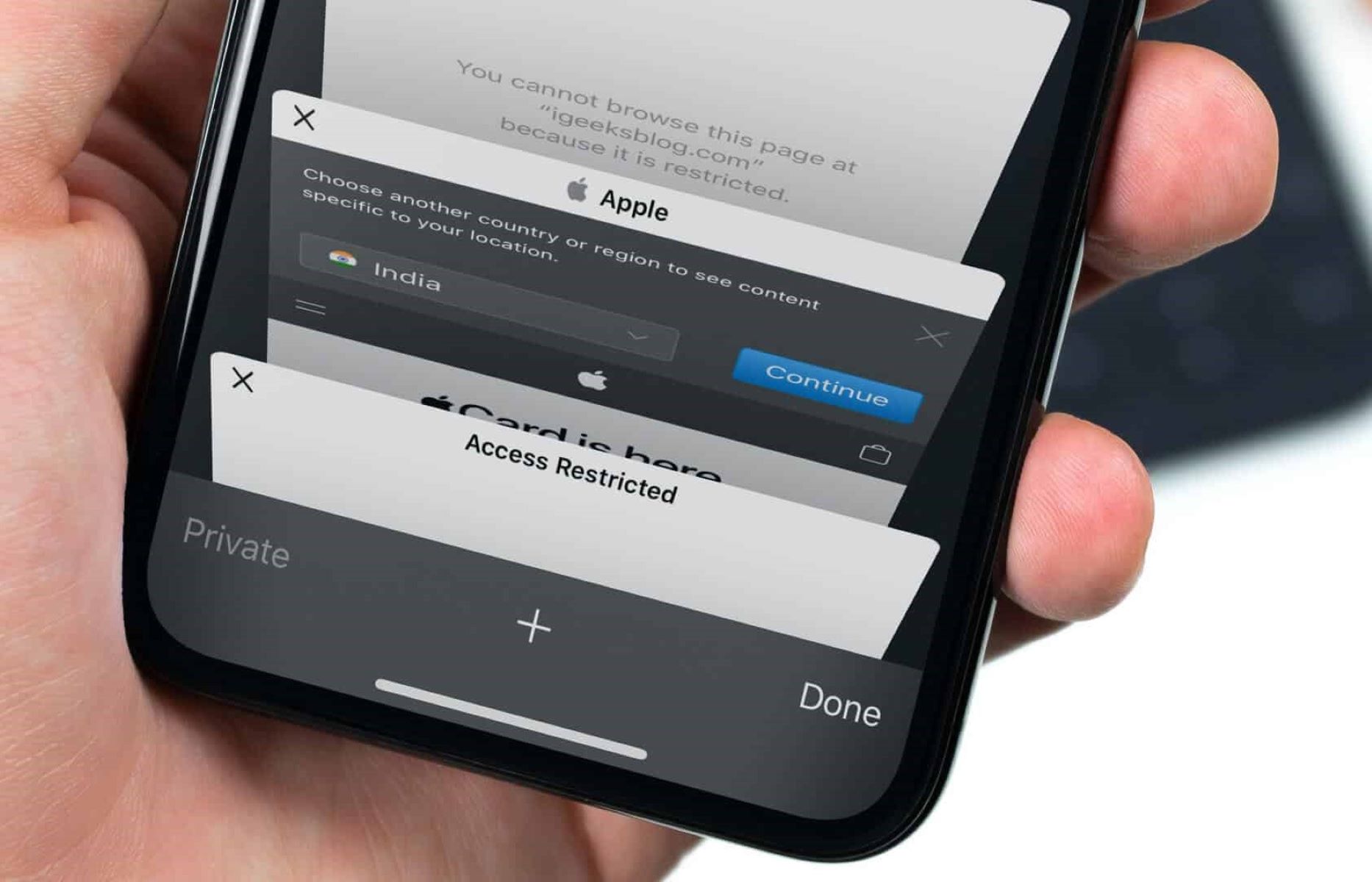
How To Open Private Browser On Safari iPhone
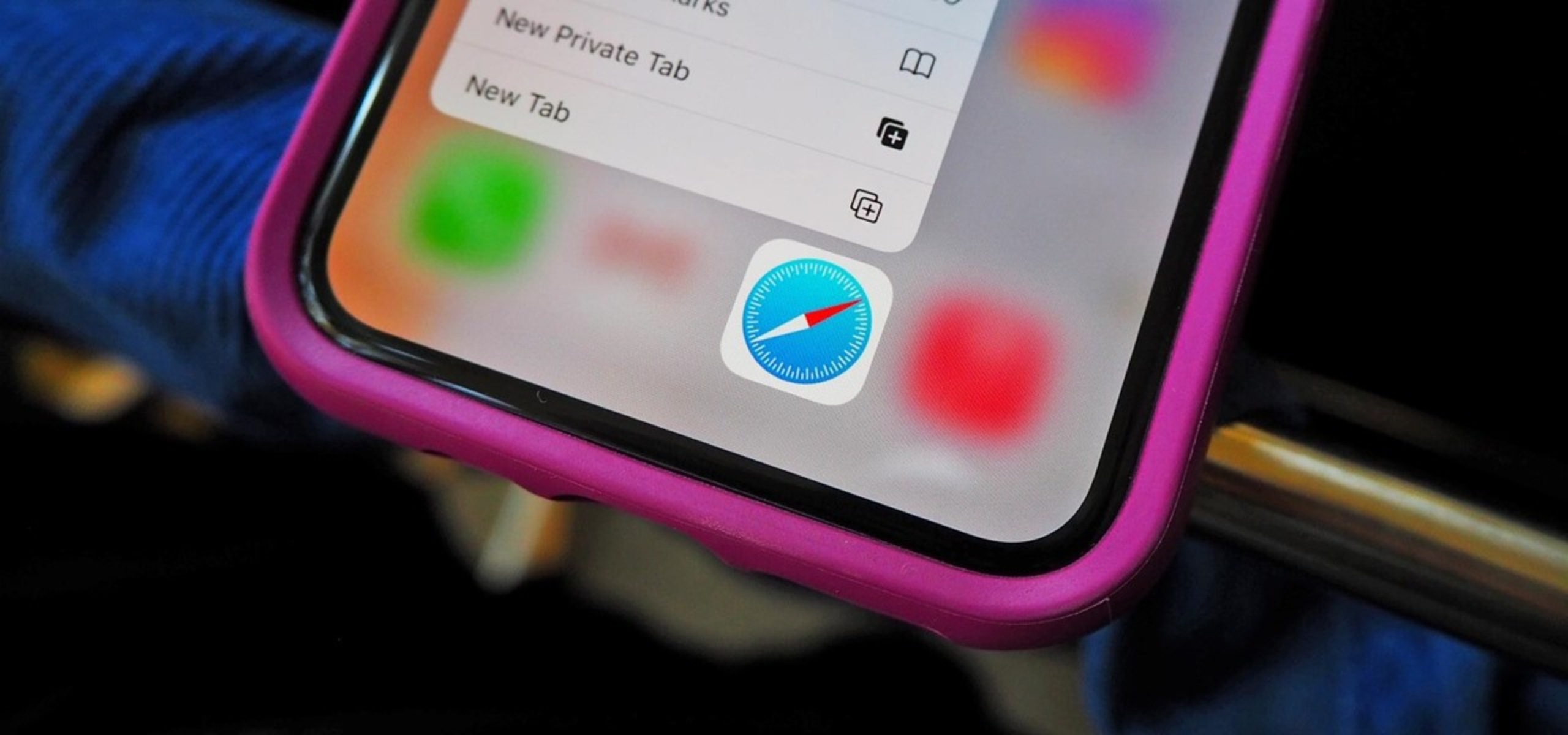
How To Change Safari Tabs Back To Normal
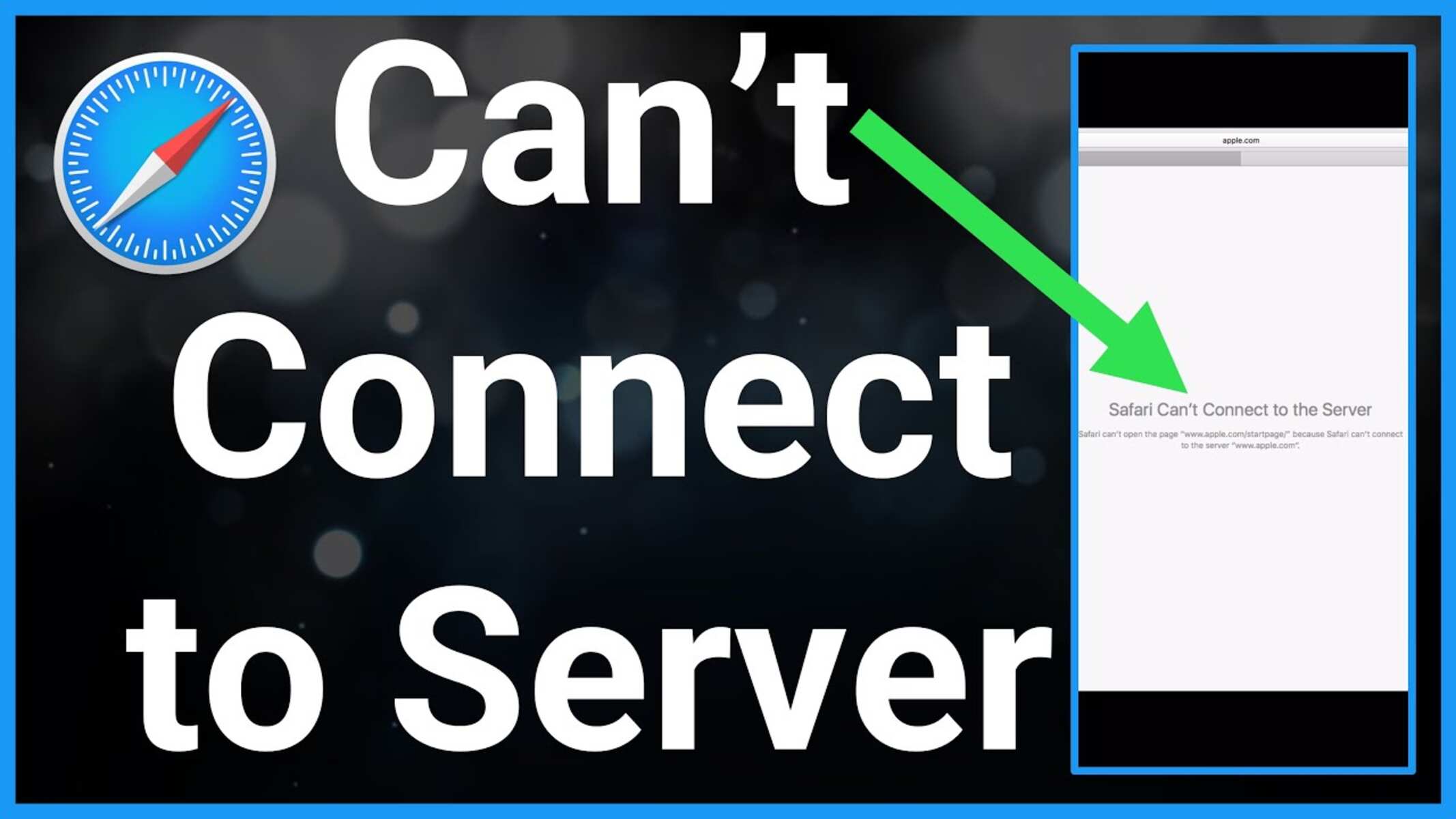
Why Does It Say Safari Cannot Connect To The Server
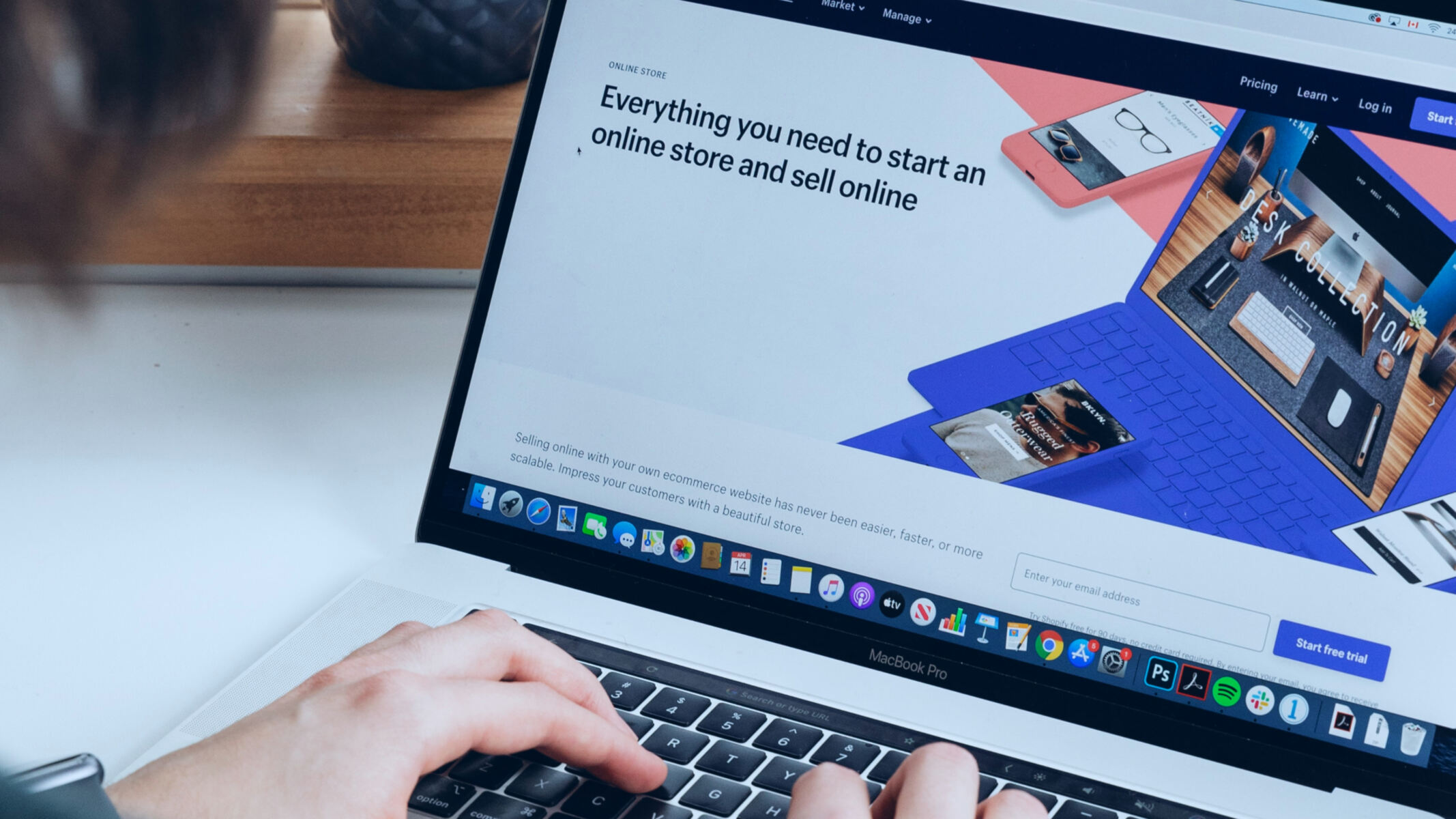
How To Shut Down Safari On A Mac
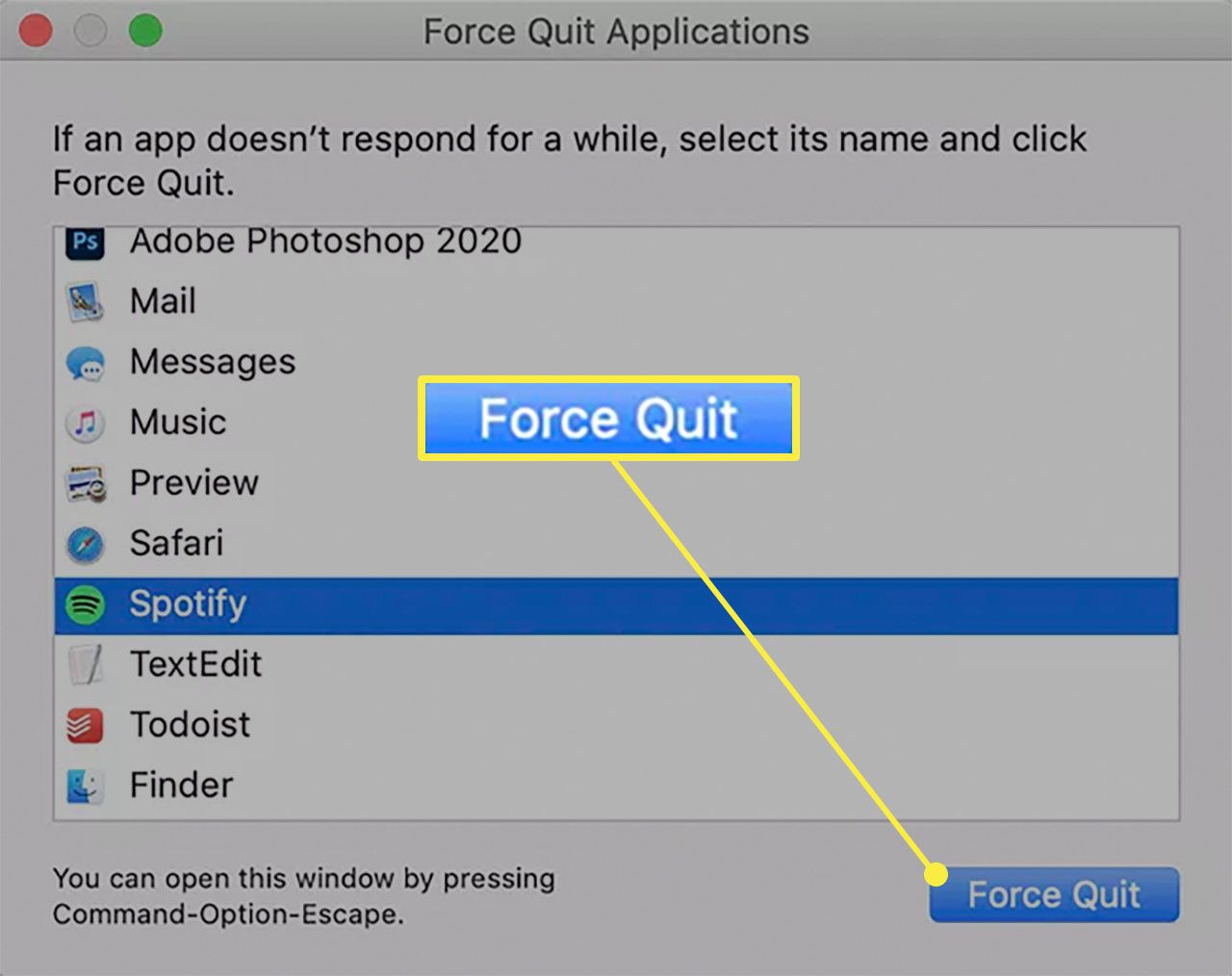
Why Won’t My Safari Quit
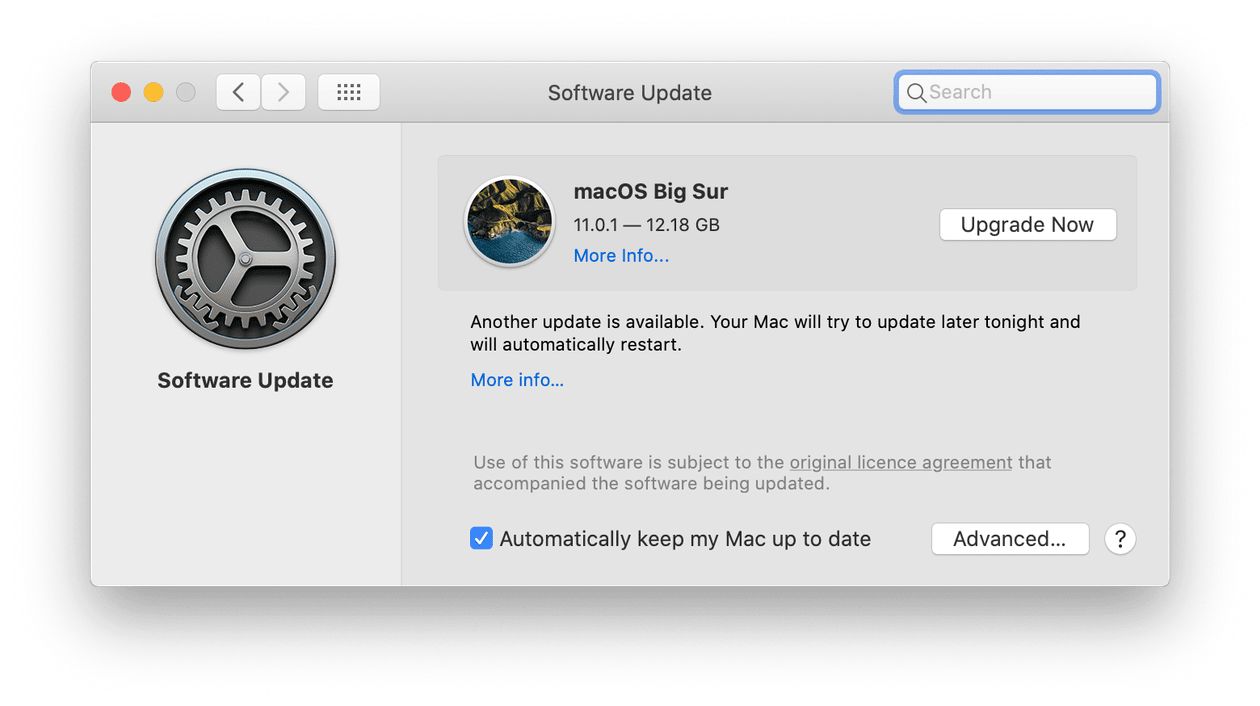
Why Won’t Safari Quit On My Mac
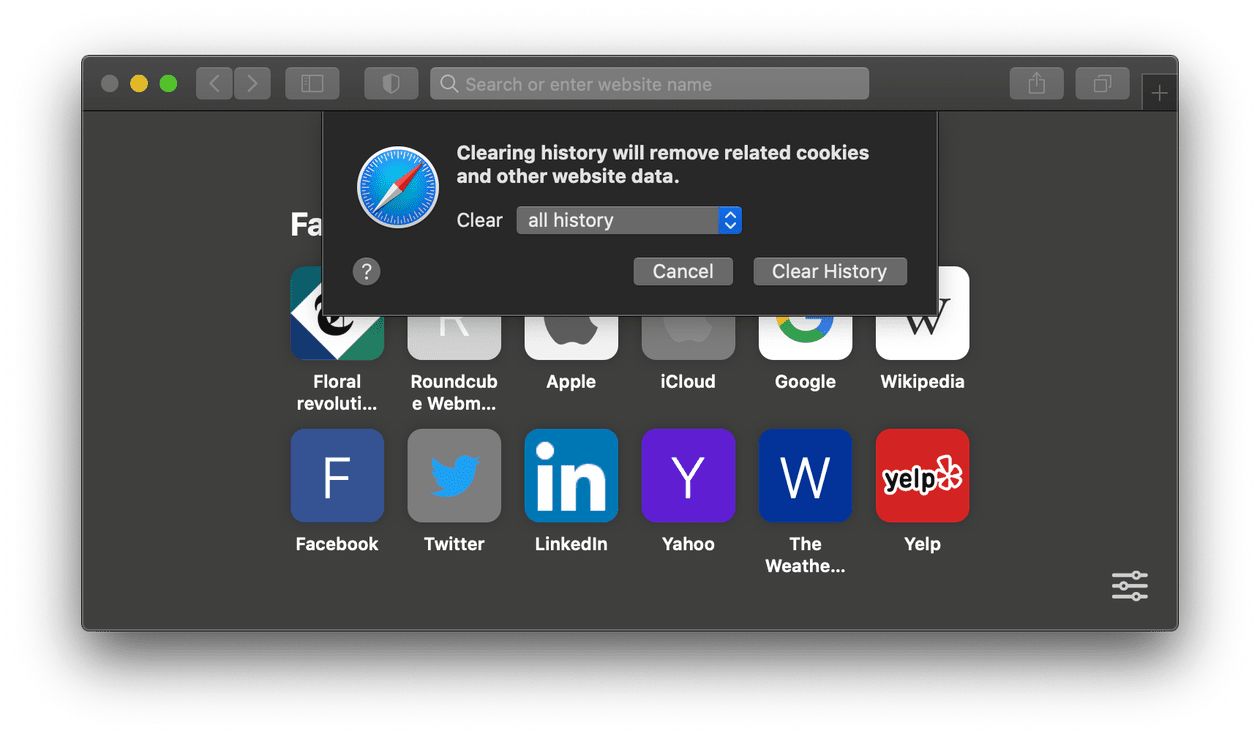
How To Force Quit Safari On A Mac
Recent stories.
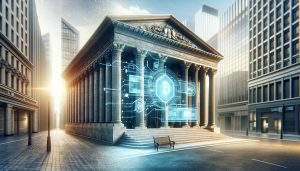
Fintechs and Traditional Banks: Navigating the Future of Financial Services
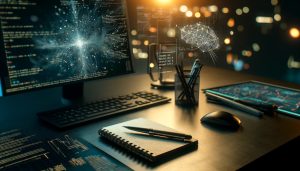
AI Writing: How It’s Changing the Way We Create Content
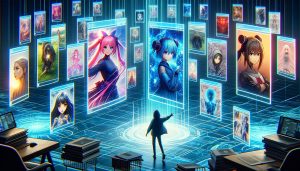
How to Know When it’s the Right Time to Buy Bitcoin
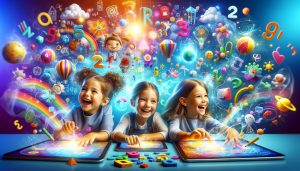
How to Sell Counter-Strike 2 Skins Instantly? A Comprehensive Guide
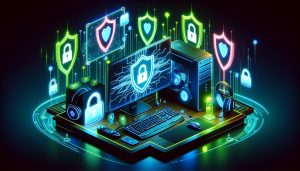
10 Proven Ways For Online Gamers To Avoid Cyber Attacks And Scams
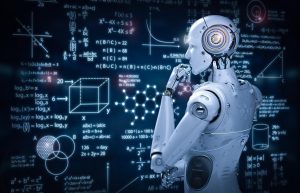
- Privacy Overview
- Strictly Necessary Cookies
This website uses cookies so that we can provide you with the best user experience possible. Cookie information is stored in your browser and performs functions such as recognising you when you return to our website and helping our team to understand which sections of the website you find most interesting and useful.
Strictly Necessary Cookie should be enabled at all times so that we can save your preferences for cookie settings.
If you disable this cookie, we will not be able to save your preferences. This means that every time you visit this website you will need to enable or disable cookies again.
- Mobile Site
- Staff Directory
- Advertise with Ars
Filter by topic
- Biz & IT
- Gaming & Culture
Front page layout
strong arm —
Is the arm version of windows ready for its close-up, checking back in with windows 11 on arm on the eve of the snapdragon x elite..
Andrew Cunningham - Apr 24, 2024 11:00 am UTC
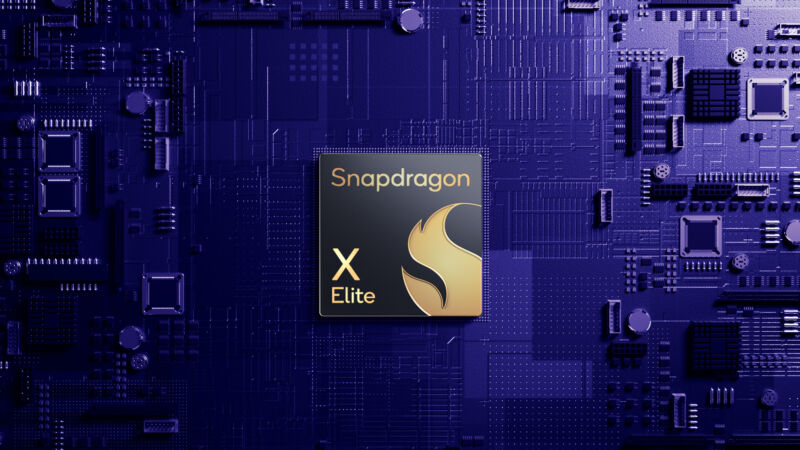
Signs point to Qualcomm’s Snapdragon X Elite processors showing up in actual, real-world, human-purchasable computers in the next couple of months after years of speculation and another year or so of hype.
For those who haven’t been following along, this will allegedly be Qualcomm’s first Arm processor for Windows PCs that does for PCs what Apple’s M-series chips did for Macs, promising both better battery life and better performance than equivalent Intel chips. This would be a departure from past Snapdragon chips for PCs, which have performed worse than (or, at best, similarly to) existing Intel options, barely improved battery life, and come with a bunch of software incompatibility problems to boot.
Early benchmarks that have trickled out look promising for the Snapdragon X. And there are other reasons to be optimistic—the Snapdragon X Elite’s design team is headed up by some of the same people who made Apple Silicon so successful in the first place.
Rumors indicate that Microsoft's flagship Surface tablet this year will switch to using Qualcomm's Arm chips exclusively rather than selling Arm and Intel versions alongside each other (an Intel Surface Pro 10 exists, but it's only sold to businesses). Microsoft has tried to make Arm Windows happen a bunch of times. But this time feels different.
A brief history of Arm Windows
The first public version of Windows to run on Arm processors was Windows RT , an Arm-compatible offshoot of Windows 8 that launched on a bare handful of devices back in late 2012.
Windows RT came with significant limitations, most notably a total inability to run traditional x86 Windows desktop apps —all apps had to come from the Microsoft Store, which was considerably more barren than it is today. There was no x86 compatibility mode at all.
That limitation may have been due in part to the limited, low-performance Arm hardware available at the time. Arm processors were still predominantly 32-bits, with slow processors and GPUs, 32 or 64GB of slow flash storage, and just 2GB of memory (at the time, 4GB was generally considered adequate for a PC, and 8GB was roomy). Even if you did have x86 app translation, translated apps would have felt awful since the Arm hardware already struggled to run the native built-in apps consistently well.
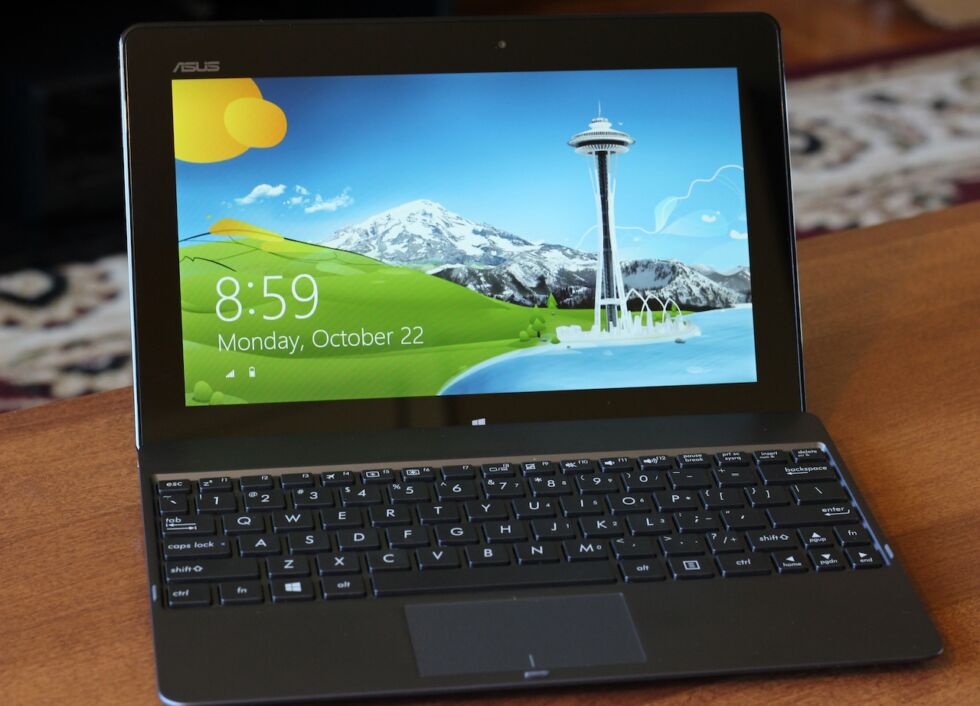
Further Reading
Windows RT died the death it deserved to die ; it never ran on many devices, and those that did had totally vanished from the market by 2015 or so. But the technical underpinnings of the operating system remain relevant today.
As detailed by then-Windows head Steven Sinofsky , Microsoft did significant work to define a hardware abstraction layer (HAL), ACPI firmware, and basic class drivers for the Arm version of Windows so that the OS could install and run as expected on a wide variety of barely standardized Arm hardware the same way it did on a thoroughly standardized x86 PC. (Compare this to Google's Wild West approach to Android, which to this day can’t install basic OS or security updates without specific intervention from chipmakers and device manufacturers).
These were building blocks Microsoft could re-use for Windows 10, which first came to Arm devices in 2017 with support for 32-bit x86 app translation. This version of Windows-on-Arm also functioned more as a technical demo than the dawn of a new era, but it did come closer to becoming what Windows-on-Arm needed to be to succeed: a drop-in replacement for the x86 version of Windows, where the two versions were largely indistinguishable for non-technical users.
The next big step down that path came in 2020 when Microsoft announced a preview of 64-bit Intel app translation for Arm PCs , though the final version ended up being exclusive to Windows 11 when it launched in late 2021 (leaving behind, incidentally, that first wave of Windows 10 Arm PCs with a Snapdragon 835 processor in them—another case where early-adopting into this ecosystem has hosed users. Developers also got an easier on-ramp to Arm when Microsoft began allowing them to mix x86 and Arm code in the same app .
That brings us to where we are now: an Arm version of Windows that still has some compatibility gaps, especially around external accessories and specialized software. But the vast majority of productivity apps and even games will now run happily on the Arm version of Windows, with no user or developer intervention required.
reader comments
Channel ars technica.

IMAGES
VIDEO
COMMENTS
$('#id-of-back-button').click(function() { window.location.href = docCookies.getItem('back-url'); }); This code sets the window location by getting the value of 'back-url'. Disclaimer: I am no professional js developer - just trying to put in my two cents from some lessons I have learned. Challenges to this answer:
The back() method of the History interface causes the browser to move back one page in the session history.. It has the same effect as calling history.go(-1).If there is no previous page, this method call does nothing. This method is asynchronous.Add a listener for the popstate event in order to determine when the navigation has completed.
Example. Create a back button on a page: <button onclick="history.back ()">Go Back</button>. The output of the code above will be: Go Back. Click on Go Back to see how it works. (Will only work if a previous page exists in your history list) Try it Yourself ».
History: scrollRestoration property. The scrollRestoration property of the History interface allows web applications to explicitly set default scroll restoration behavior on history navigation.
The Window.history read-only property returns a reference to the History object, which provides an interface for manipulating the browser session history (pages visited in the tab or frame that the current page is loaded in).. See Manipulating the browser history for examples and details. In particular, that article explains security features of the pushState() and replaceState() methods that ...
To move back one page (the equivalent of calling back()): window.history.go(-1); To move forward a page, just like calling forward(): window.history.go(1); Similarly, you can move forward 2 pages by passing 2, and so forth. You can determine the number of pages in the history stack by looking at the value of the length property:
The Window.history read-only property returns a reference to the History object, which provides an interface for manipulating the browser session history (pages visited in the tab or frame that the current page is loaded in).. See Manipulating the browser history for examples and details. In particular, that article explains security features of the pushState() and replaceState() methods that ...
How to Reopen Closed Safari Tabs and Windows and Access Past History. Quickly get back to where you were. Undo a closed tab: Go to Edit > Undo Close Tab, press Command + Z, or click and hold the plus sign to the right of the Tabs bar. Restore a closed window: Go to History > Reopen Last Closed Window. Or, History > Reopen All Windows From Last ...
In the Safari app on your Mac, choose History > Clear History, then click the pop-up menu. Choose how far back you want your browsing history cleared. When you clear your history, Safari removes data it saves as a result of your browsing, including: In Safari on your Mac, remove all records of your browsing history for a period of time you choose.
window. history.forward(); Moving to a specific point in history. You can use the go() method to load a specific page from session history, identified by its relative position to the current page (with the current page being, of course, relative index 0).. To move back one page (the equivalent of calling back()):
Use the search bar to find specific websites. Enter your text in the Search bar. A list of matching results from your history will appear. Click a site to load it in Safari. 5. To clear your history, click the "History" menu. Click Clear History…. [2] Select a time frame from the drop-down menu, then click Clear History.
In the Safari app on your Mac, choose History > Show All History. Type in the Search field at the top-right corner of the window. Safari uses iCloud to keep your browsing history the same on your iPhone, iPad, iPod touch and Mac computers that have Safari turned on in iCloud preferences. On your iPhone, iPad or iPod touch, go to Settings ...
Losing your browsing history on Safari can be a frustrating experience, especially when you need to revisit a website or retrieve important information. Whether it's due to accidental deletion, software issues, or other unforeseen circumstances, the good news is that there are several methods you can explore to recover your Safari history.
Delete a website from your history. Block cookies. Use content blockers. Delete history, cache, and cookies. Go to Settings > Safari. Tap Clear History and Website Data. Clearing your history, cookies, and browsing data from Safari won't change your AutoFill information. When there's no history or website data to clear, the button to clear it ...
The back() and go() methods are supported in all major browsers. History is one of the sub-objects of Windows, and an interface to the history stored by your browser. Properties and methods apply to the object history of windows or to each frame in the window. history.go(0) acts like pressing the reload button.
In the Safari Preferences window, navigate to the "History" tab and click on "Clear History." You can choose the time range for which you want to clear the history and then click "Clear History." Restart Safari: Once you've cleared the cache and history, it's a good idea to restart Safari to ensure that the changes take effect.
After struggling with this for a few days, it turns out that you can't do a window.location.reload() after a window.history.go(-2), because the code stops running after the window.history.go(-2).Also the html spec basically views a history.go(-2) to the the same as hitting the back button and should retrieve the page as it was instead of as it now may be.
A brief history of Arm Windows The first public version of Windows to run on Arm processors was Windows RT , an Arm-compatible offshoot of Windows 8 that launched on a bare handful of devices back ...
History.back() メソッドは、ブラウザーにセッション履歴内で 1 つ前のページに戻らせます。 これは history.go(-1) を呼び出すのと同じ効果があります。 1 つ前のページがない場合、このメソッド呼び出しは何もしません。 このメソッドは非同期です。 移動が完了したときを検知したい場合は popstate ...
Suppose you set window.history.scrollRestoration = "manual" on a webpage. In Chrome/Firefox. Whenever you click an anchor, the page scrolls to the linked element's position, and whenever you go back/forward through history, the scroll position remains the same but the fragment part of the url is updated (#sectionXYZ). In Safari